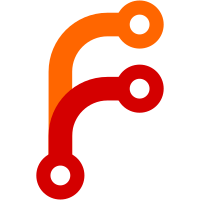
Co-authored-by: Omar López <zomars@me.com> Co-authored-by: Peer Richelsen <peeroke@gmail.com>
180 lines
4.2 KiB
Text
180 lines
4.2 KiB
Text
---
|
|
title: Embed
|
|
---
|
|
|
|
# Embed
|
|
|
|
The Embed allows your website visitors to book a meeting with you directly from your website.
|
|
|
|
## Install on any website
|
|
|
|
TODO: Mention possibility of installation through tag managers as well
|
|
|
|
- _Step-1._ Install the Vanilla JS Snippet
|
|
|
|
```javascript
|
|
(function (C, A, L) {
|
|
let p = function (a, ar) {
|
|
a.q.push(ar);
|
|
};
|
|
let d = C.document;
|
|
C.Cal =
|
|
C.Cal ||
|
|
function () {
|
|
let cal = C.Cal;
|
|
let ar = arguments;
|
|
if (!cal.loaded) {
|
|
cal.ns = {};
|
|
cal.q = cal.q || [];
|
|
d.head.appendChild(d.createElement("script")).src = A;
|
|
cal.loaded = true;
|
|
}
|
|
if (ar[0] === L) {
|
|
const api = function () {
|
|
p(api, arguments);
|
|
};
|
|
const namespace = ar[1];
|
|
api.q = api.q || [];
|
|
typeof namespace === "string" ? (cal.ns[namespace] = api) && p(api, ar) : p(cal, ar);
|
|
return;
|
|
}
|
|
p(cal, ar);
|
|
};
|
|
})(window, "https://cal.com/embed.js", "init");
|
|
```
|
|
|
|
- _Step-2_. Initialize it
|
|
|
|
```javascript
|
|
Cal("init)
|
|
```
|
|
|
|
## Install with a Framework
|
|
|
|
### embed-react
|
|
|
|
It provides a react component `<Cal>` that can be used to show the embed inline at that place.
|
|
|
|
```bash
|
|
yarn add @calcom/embed-react
|
|
```
|
|
|
|
### Any XYZ Framework
|
|
|
|
You can use Vanilla JS Snippet to install
|
|
|
|
## Popular ways in which you can embed on your website
|
|
|
|
Assuming that you have followed the steps of installing and initializing the snippet, you can add show the embed in following ways:
|
|
|
|
### Inline
|
|
|
|
Show the embed inline inside a container element. It would take the width and height of the container element.
|
|
|
|
<details>
|
|
<summary>_Vanilla JS_</summary>
|
|
|
|
```javascript
|
|
Cal("inline", {
|
|
elementOrSelector: "Your Embed Container Selector Path", // You can also provide an element directly
|
|
calLink: "jane", // The link that you want to embed. It would open https://cal.com/jane in embed
|
|
config: {
|
|
name: "John Doe", // Prefill Name
|
|
email: "johndoe@gmail.com", // Prefill Email
|
|
notes: "Test Meeting", // Prefill Notes
|
|
guests: ["janedoe@gmail.com", "test@gmail.com"], // Prefill Guests
|
|
theme: "dark", // "dark" or "light" theme
|
|
},
|
|
});
|
|
```
|
|
|
|
</details>
|
|
|
|
####
|
|
|
|
<details>
|
|
<summary>_React_</summary>
|
|
|
|
```jsx
|
|
import Cal from "@calcom/embed-react";
|
|
|
|
const MyComponent = () => (
|
|
<Cal
|
|
calLink="pro"
|
|
config={{
|
|
name: "John Doe",
|
|
email: "johndoe@gmail.com",
|
|
notes: "Test Meeting",
|
|
guests: ["janedoe@gmail.com"],
|
|
theme: "dark",
|
|
}}
|
|
/>
|
|
);
|
|
```
|
|
|
|
</details>
|
|
|
|
### Popup on any existing element
|
|
|
|
To show the embed as a popup on clicking an element, add `data-cal-link` attribute to the element.
|
|
|
|
<details>
|
|
|
|
<summary>Vanilla JS</summary>
|
|
|
|
To show the embed as a popup on clicking an element, simply add `data-cal-link` attribute to the element.
|
|
|
|
<button data-cal-link="jane" data-cal-config="A valid config JSON"></button>
|
|
</details>
|
|
|
|
<details>
|
|
<summary>React</summary>
|
|
```jsx
|
|
import "@calcom/embed-react";
|
|
|
|
const MyComponent = ()=> {
|
|
return <button data-cal-link="jane" data-cal-config='A valid config JSON'></button>
|
|
}
|
|
|
|
````
|
|
|
|
</details>
|
|
### Full Screen
|
|
|
|
## Supported Instructions
|
|
|
|
Consider an instruction as a function with that name and that would be called with the given arguments.
|
|
|
|
### `inline`
|
|
|
|
Appends embed inline as the child of the element.
|
|
|
|
```javascript
|
|
Cal("inline", { elementOrSelector, calLink });
|
|
````
|
|
|
|
- `elementOrSelector` - Give it either a valid CSS selector or an HTMLElement instance directly
|
|
|
|
- `calLink` - Cal Link that you want to embed e.g. john. Just give the username. No need to give the full URL [https://cal.com/john](). It makes it easy to configure the calendar host once and use as many links you want with just usernames
|
|
|
|
### `ui`
|
|
|
|
Configure UI for embed. Make it look part of your webpage.
|
|
|
|
```javascript
|
|
Cal("inline", { styles });
|
|
```
|
|
|
|
- `styles` - It supports styling for `body` and `eventTypeListItem`. Right now we support just background on these two.
|
|
|
|
### preload
|
|
|
|
Usage:
|
|
|
|
If you want to open cal link on some action. Make it pop open instantly by preloading it.
|
|
|
|
```javascript
|
|
Cal("preload", { calLink });
|
|
```
|
|
|
|
- `calLink` - Cal Link that you want to embed e.g. john. Just give the username. No need to give the full URL [https://cal.com/john]()
|