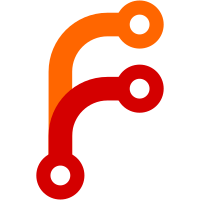
* fix: adds new isBrowserLocal24h timeFormat util, uses in BookingPage * fix: adds new time format locale detector in available times * fix: removes 24h clock from availabletimes * chore: move timeFormat to lib util, add to payment page * chore: remove commented out is24h * fix: adds timeFormat to success page * fix: adds timeFormat to cancel page * fix: adds timeFormat to video meeting ended/not started pages * fix: removes added typo in success page * fix: reverts back to use of is24h Switch in available times / time options, renames timeFormat to detectBrowserTimeFormat to avoid collisions * fix: moves use uf isBrowserLocal24h() to clock util initClock() itself, by calling it only if no localStorage settings are set * chore: move back timeFormat props to line it was so no change * chore: remove empty line in timeOptions * chore: move back timeFormat where it was in TimeOptions props * chore: add back empty line before selectedTimeZone return * fix: reverts back to use of is24h in payments page * feat: adds browser locale as default in payment page in case there's no settings set by the customer * feat: adds browser locale as default in success page * fix: deconstruct props so eslint passes * fix: lint issue for extra empty line in meeting-ended uid page Co-authored-by: Agusti Fernandez <git@agusti.me> Co-authored-by: kodiakhq[bot] <49736102+kodiakhq[bot]@users.noreply.github.com>
55 lines
1.6 KiB
TypeScript
55 lines
1.6 KiB
TypeScript
// handles logic related to user clock display using 24h display / timeZone options.
|
|
import dayjs from "dayjs";
|
|
import timezone from "dayjs/plugin/timezone";
|
|
import utc from "dayjs/plugin/utc";
|
|
|
|
import { isBrowserLocale24h } from "./timeFormat";
|
|
|
|
dayjs.extend(utc);
|
|
dayjs.extend(timezone);
|
|
|
|
interface TimeOptions {
|
|
is24hClock: boolean;
|
|
inviteeTimeZone: string;
|
|
}
|
|
|
|
const timeOptions: TimeOptions = {
|
|
is24hClock: false,
|
|
inviteeTimeZone: "",
|
|
};
|
|
|
|
const isInitialized = false;
|
|
|
|
const initClock = () => {
|
|
if (typeof localStorage === "undefined" || isInitialized) {
|
|
return;
|
|
}
|
|
// This only sets browser locale if there's no preference on localStorage.
|
|
if (!localStorage || !localStorage.getItem("timeOption.is24hClock")) set24hClock(isBrowserLocale24h());
|
|
timeOptions.is24hClock = localStorage.getItem("timeOption.is24hClock") === "true";
|
|
timeOptions.inviteeTimeZone = localStorage.getItem("timeOption.preferredTimeZone") || dayjs.tz.guess();
|
|
};
|
|
|
|
const is24h = (is24hClock?: boolean) => {
|
|
initClock();
|
|
if (typeof is24hClock !== "undefined") set24hClock(is24hClock);
|
|
return timeOptions.is24hClock;
|
|
};
|
|
|
|
const set24hClock = (is24hClock: boolean) => {
|
|
localStorage.setItem("timeOption.is24hClock", is24hClock.toString());
|
|
timeOptions.is24hClock = is24hClock;
|
|
};
|
|
|
|
function setTimeZone(selectedTimeZone: string) {
|
|
localStorage.setItem("timeOption.preferredTimeZone", selectedTimeZone);
|
|
timeOptions.inviteeTimeZone = selectedTimeZone;
|
|
}
|
|
|
|
const timeZone = (selectedTimeZone?: string) => {
|
|
initClock();
|
|
if (selectedTimeZone) setTimeZone(selectedTimeZone);
|
|
return timeOptions.inviteeTimeZone;
|
|
};
|
|
|
|
export { is24h, timeZone };
|