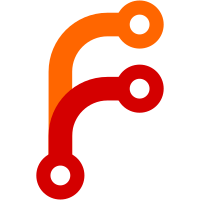
* [CAL-770] add new integration architecture revamp * Type fixes * Type fixes * [CAL-770] Remove tsconfig.tsbuildinfo * [CAL-770] add integration test * Improve google calendar test integration * Remove console.log * Change response any to void in the deleteEvent method * Remove unnecesary const * Add tsconfig.tsbuildinfo to the .gitignore * Remove process env variables as const Co-authored-by: Edward Fernández <edwardfernandez@Edwards-Mac-mini.local> Co-authored-by: kodiakhq[bot] <49736102+kodiakhq[bot]@users.noreply.github.com> Co-authored-by: zomars <zomars@me.com> Co-authored-by: Edward Fernandez <edward.fernandez@rappi.com>
63 lines
1.5 KiB
TypeScript
63 lines
1.5 KiB
TypeScript
import { compile } from "handlebars";
|
|
|
|
import { CalendarEvent } from "@lib/integrations/calendar/interfaces/Calendar";
|
|
|
|
type ContentType = "application/json" | "application/x-www-form-urlencoded";
|
|
|
|
function applyTemplate(template: string, data: Omit<CalendarEvent, "language">, contentType: ContentType) {
|
|
const compiled = compile(template)(data);
|
|
if (contentType === "application/json") {
|
|
return jsonParse(compiled);
|
|
}
|
|
return compiled;
|
|
}
|
|
|
|
function jsonParse(jsonString: string) {
|
|
try {
|
|
return JSON.parse(jsonString);
|
|
} catch (e) {
|
|
// don't do anything.
|
|
}
|
|
return false;
|
|
}
|
|
|
|
const sendPayload = async (
|
|
triggerEvent: string,
|
|
createdAt: string,
|
|
subscriberUrl: string,
|
|
data: Omit<CalendarEvent, "language"> & { metadata?: { [key: string]: string } },
|
|
template?: string | null
|
|
) => {
|
|
if (!subscriberUrl || !data) {
|
|
throw new Error("Missing required elements to send webhook payload.");
|
|
}
|
|
|
|
const contentType =
|
|
!template || jsonParse(template) ? "application/json" : "application/x-www-form-urlencoded";
|
|
|
|
const body = template
|
|
? applyTemplate(template, data, contentType)
|
|
: JSON.stringify({
|
|
triggerEvent: triggerEvent,
|
|
createdAt: createdAt,
|
|
payload: data,
|
|
});
|
|
|
|
const response = await fetch(subscriberUrl, {
|
|
method: "POST",
|
|
headers: {
|
|
"Content-Type": contentType,
|
|
},
|
|
body,
|
|
});
|
|
|
|
const text = await response.text();
|
|
|
|
return {
|
|
ok: response.ok,
|
|
status: response.status,
|
|
message: text,
|
|
};
|
|
};
|
|
|
|
export default sendPayload;
|