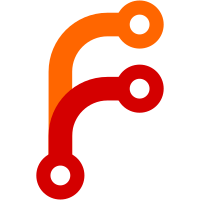
* added prisma models and migration, minor webhook init --WIP * --WIP * --WIP * added radix-checkbox and other webhook additions --WIP * added API connections and other modifications --WIP * --WIP * replaced checkbox with toggle --WIP * updated to use Dialog instead of modal --WIP * fixed API and other small fixes -WIP * created a dummy hook for test --WIP * replaced static hook with dynamic hooks * yarn lock conflict quickfix * added cancel event hook and other minor additions --WIP * minor improvements --WIP * added more add-webhook flow items--WIP * updated migration to have alter table for eventType * many ui/ux fixes, logic fixes and action fixes --WIP * bugfix for incorrect webhook filtering * some more fixes, edit webhook --WIP * removed redundant checkbox * more bugfixes and edit-webhook flow --WIP * more build and lint fixes * --WIP * more fixes and added toast notif --WIP * --updated iconButton * clean-up * fixed enabled check in edit webhook * another fix * fixed edit webhook bug * added await to payload lambda * wrapped payload call in promise * fixed cancel/uid CTA alignment * --requested changes --removed eventType relationship * Adds missing migration * Fixes missing daysjs plugin and type fixes * Adds failsafe for webhooks * Adds missing dayjs utc plugins * Fixed schema and migrations * Updates webhooks query Co-authored-by: Peer Richelsen <peeroke@gmail.com> Co-authored-by: kodiakhq[bot] <49736102+kodiakhq[bot]@users.noreply.github.com> Co-authored-by: Omar López <zomars@me.com>
57 lines
1.4 KiB
TypeScript
57 lines
1.4 KiB
TypeScript
import type { NextApiRequest, NextApiResponse } from "next";
|
|
import { getSession } from "next-auth/client";
|
|
|
|
import prisma from "@lib/prisma";
|
|
|
|
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
|
|
const session = await getSession({ req: req });
|
|
if (!session) {
|
|
return res.status(401).json({ message: "Not authenticated" });
|
|
}
|
|
|
|
// GET /api/webhook/{hook}
|
|
const webhooks = await prisma.webhook.findFirst({
|
|
where: {
|
|
id: String(req.query.hook),
|
|
userId: session.user.id,
|
|
},
|
|
});
|
|
if (req.method === "GET") {
|
|
return res.status(200).json({ webhooks: webhooks });
|
|
}
|
|
|
|
// DELETE /api/webhook/{hook}
|
|
if (req.method === "DELETE") {
|
|
await prisma.webhook.delete({
|
|
where: {
|
|
id: String(req.query.hook),
|
|
},
|
|
});
|
|
return res.status(200).json({});
|
|
}
|
|
|
|
if (req.method === "PATCH") {
|
|
const webhook = await prisma.webhook.findUnique({
|
|
where: {
|
|
id: req.query.hook as string,
|
|
},
|
|
});
|
|
|
|
if (!webhook) {
|
|
return res.status(404).json({ message: "Invalid Webhook" });
|
|
}
|
|
|
|
await prisma.webhook.update({
|
|
where: {
|
|
id: req.query.hook as string,
|
|
},
|
|
data: {
|
|
subscriberUrl: req.body.subscriberUrl,
|
|
eventTriggers: req.body.eventTriggers,
|
|
active: req.body.enabled,
|
|
},
|
|
});
|
|
|
|
return res.status(200).json({ message: "Webhook updated successfully" });
|
|
}
|
|
}
|