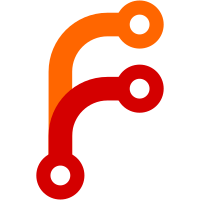
* WIP bookings page ui changes, created api endpoint * Ui changes mobile/desktop * Added translations * Fix lib import and common names * WIP reschedule * WIP * Save wip * [WIP] builder and class for CalendarEvent, email for attende * update rescheduled emails, booking view and availability page view * Working version reschedule * Fix for req.user as array * Added missing translation and refactor dialog to self component * Test for reschedule * update on types * Update lib no required * Update type on createBooking * fix types * remove preview stripe sub * remove unused file * remove unused import * Fix reschedule test * Refactor and cleaning up code * Email reschedule title fixes * Adding calendar delete and recreate placeholder of cancelled * Add translation * Removed logs, notes, fixed types * Fixes process.env types * Use strict compare * Fixes type inference * Type fixing is my middle name * Update apps/web/components/booking/BookingListItem.tsx * Update apps/web/components/dialog/RescheduleDialog.tsx * Update packages/core/builders/CalendarEvent/director.ts * Update apps/web/pages/success.tsx * Updates rescheduling labels * Update packages/core/builders/CalendarEvent/builder.ts * Type fixes * Update packages/core/builders/CalendarEvent/builder.ts * Only validating input blocked once * E2E fixes * Stripe tests fixes Co-authored-by: Peer Richelsen <peer@cal.com> Co-authored-by: zomars <zomars@me.com>
51 lines
1.6 KiB
TypeScript
51 lines
1.6 KiB
TypeScript
import { PrismaClient } from "@prisma/client";
|
|
|
|
async function middleware(prisma: PrismaClient) {
|
|
/***********************************/
|
|
/* SOFT DELETE MIDDLEWARE */
|
|
/***********************************/
|
|
prisma.$use(async (params, next) => {
|
|
// Check incoming query type
|
|
|
|
if (params.model === "BookingReference") {
|
|
if (params.action === "delete") {
|
|
// Delete queries
|
|
// Change action to an update
|
|
params.action = "update";
|
|
params.args["data"] = { deleted: true };
|
|
}
|
|
if (params.action === "deleteMany") {
|
|
console.log("deletingMany");
|
|
// Delete many queries
|
|
params.action = "updateMany";
|
|
if (params.args.data !== undefined) {
|
|
params.args.data["deleted"] = true;
|
|
} else {
|
|
params.args["data"] = { deleted: true };
|
|
}
|
|
}
|
|
if (params.action === "findUnique") {
|
|
// Change to findFirst - you cannot filter
|
|
// by anything except ID / unique with findUnique
|
|
params.action = "findFirst";
|
|
// Add 'deleted' filter
|
|
// ID filter maintained
|
|
params.args.where["deleted"] = null;
|
|
}
|
|
if (params.action === "findMany" || params.action === "findFirst") {
|
|
// Find many queries
|
|
if (params.args.where !== undefined) {
|
|
if (params.args.where.deleted === undefined) {
|
|
// Exclude deleted records if they have not been explicitly requested
|
|
params.args.where["deleted"] = null;
|
|
}
|
|
} else {
|
|
params.args["where"] = { deleted: null };
|
|
}
|
|
}
|
|
}
|
|
return next(params);
|
|
});
|
|
}
|
|
|
|
export default middleware;
|