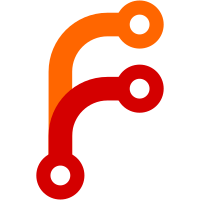
* WIP bookings page ui changes, created api endpoint * Ui changes mobile/desktop * Added translations * Fix lib import and common names * WIP reschedule * WIP * Save wip * [WIP] builder and class for CalendarEvent, email for attende * update rescheduled emails, booking view and availability page view * Working version reschedule * Fix for req.user as array * Added missing translation and refactor dialog to self component * Test for reschedule * update on types * Update lib no required * Update type on createBooking * fix types * remove preview stripe sub * remove unused file * remove unused import * Fix reschedule test * Refactor and cleaning up code * Email reschedule title fixes * Adding calendar delete and recreate placeholder of cancelled * Add translation * Removed logs, notes, fixed types * Fixes process.env types * Use strict compare * Fixes type inference * Type fixing is my middle name * Update apps/web/components/booking/BookingListItem.tsx * Update apps/web/components/dialog/RescheduleDialog.tsx * Update packages/core/builders/CalendarEvent/director.ts * Update apps/web/pages/success.tsx * Updates rescheduling labels * Update packages/core/builders/CalendarEvent/builder.ts * Type fixes * Update packages/core/builders/CalendarEvent/builder.ts * Only validating input blocked once * E2E fixes * Stripe tests fixes Co-authored-by: Peer Richelsen <peer@cal.com> Co-authored-by: zomars <zomars@me.com>
80 lines
1.8 KiB
TypeScript
80 lines
1.8 KiB
TypeScript
import { Booking } from "@prisma/client";
|
|
import dayjs from "dayjs";
|
|
import utc from "dayjs/plugin/utc";
|
|
import short from "short-uuid";
|
|
import { v5 as uuidv5, v4 as uuidv4 } from "uuid";
|
|
|
|
dayjs.extend(utc);
|
|
|
|
const translator = short();
|
|
|
|
const TestUtilCreateBookingOnUserId = async (
|
|
userId: number,
|
|
username: string,
|
|
eventTypeId: number,
|
|
{ confirmed = true, rescheduled = false, paid = false, status = "ACCEPTED" }: Partial<Booking>
|
|
) => {
|
|
const startDate = dayjs().add(1, "day").toDate();
|
|
const seed = `${username}:${dayjs(startDate).utc().format()}:${new Date().getTime()}`;
|
|
const uid = translator.fromUUID(uuidv5(seed, uuidv5.URL));
|
|
return await prisma?.booking.create({
|
|
data: {
|
|
uid: uid,
|
|
title: "30min",
|
|
startTime: startDate,
|
|
endTime: dayjs().add(1, "day").add(30, "minutes").toDate(),
|
|
user: {
|
|
connect: {
|
|
id: userId,
|
|
},
|
|
},
|
|
attendees: {
|
|
create: {
|
|
email: "attendee@example.com",
|
|
name: "Attendee Example",
|
|
timeZone: "Europe/London",
|
|
},
|
|
},
|
|
eventType: {
|
|
connect: {
|
|
id: eventTypeId,
|
|
},
|
|
},
|
|
confirmed,
|
|
rescheduled,
|
|
paid,
|
|
status,
|
|
},
|
|
select: {
|
|
id: true,
|
|
uid: true,
|
|
user: true,
|
|
},
|
|
});
|
|
};
|
|
|
|
const TestUtilCreatePayment = async (
|
|
bookingId: number,
|
|
{ success = false, refunded = false }: { success?: boolean; refunded?: boolean }
|
|
) => {
|
|
return await prisma?.payment.create({
|
|
data: {
|
|
uid: uuidv4(),
|
|
amount: 20000,
|
|
fee: 160,
|
|
currency: "usd",
|
|
success,
|
|
refunded,
|
|
type: "STRIPE",
|
|
data: {},
|
|
externalId: "DEMO_PAYMENT_FROM_DB",
|
|
booking: {
|
|
connect: {
|
|
id: bookingId,
|
|
},
|
|
},
|
|
},
|
|
});
|
|
};
|
|
|
|
export { TestUtilCreateBookingOnUserId, TestUtilCreatePayment };
|